Using Multichannel and Speaker Diarization
Learn how Multichannel transcription and Speaker Diarization work, what their outputs look like, when to use each feature, and how you can implement them.

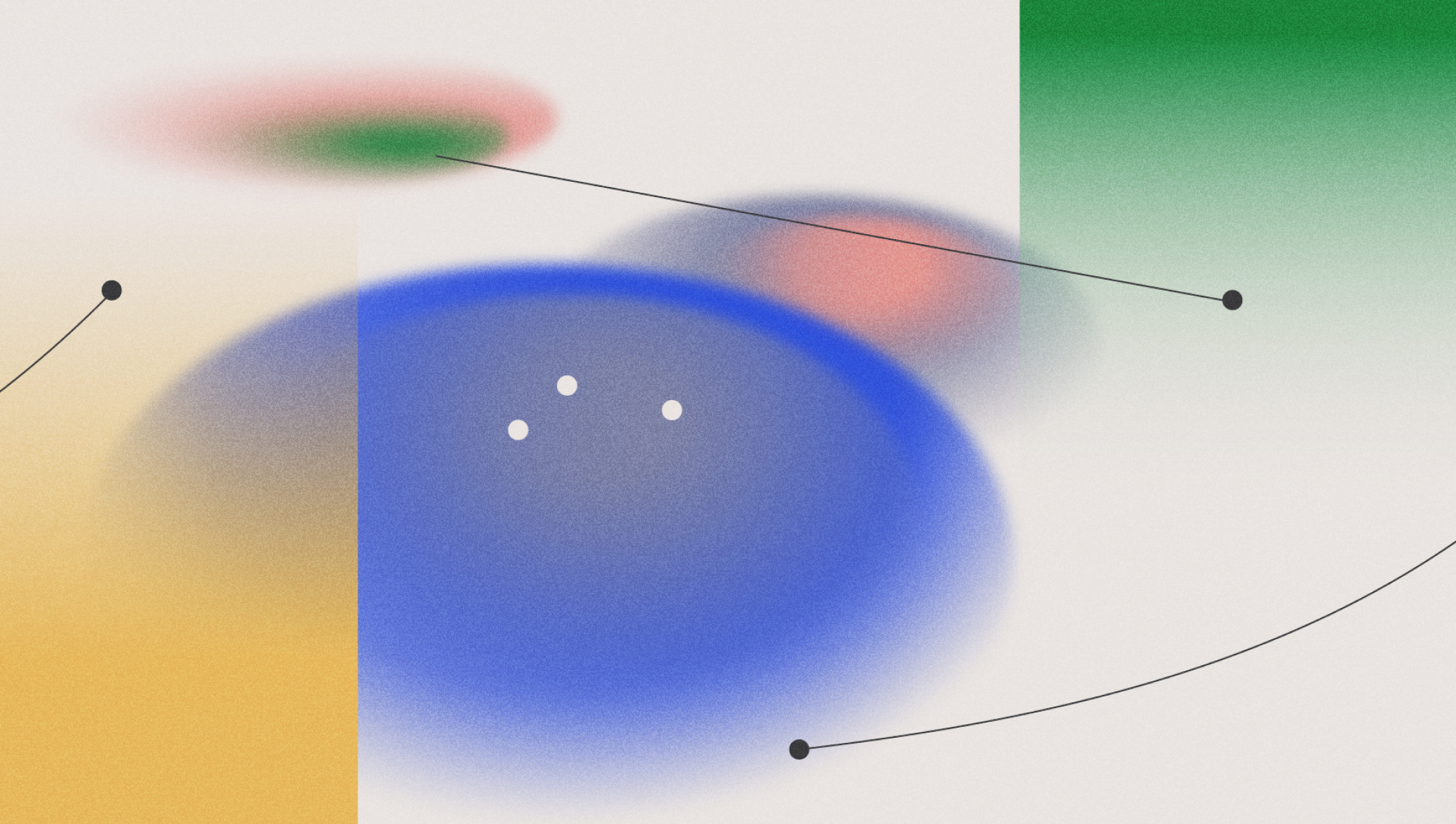

When working with audio recordings that feature multiple speakers, separating and identifying each participant is a crucial step in producing accurate and organized transcriptions. Two techniques that make this possible are Multichannel transcription and Speaker Diarization.
Multichannel transcription, also known as channel diarization, processes audio recordings with separate channels for each speaker, making it easier to isolate individual contributions. Speaker Diarization, on the other hand, focuses on distinguishing speakers in single-channel recordings. Both methods help create structured transcripts that are easy to analyze and use.
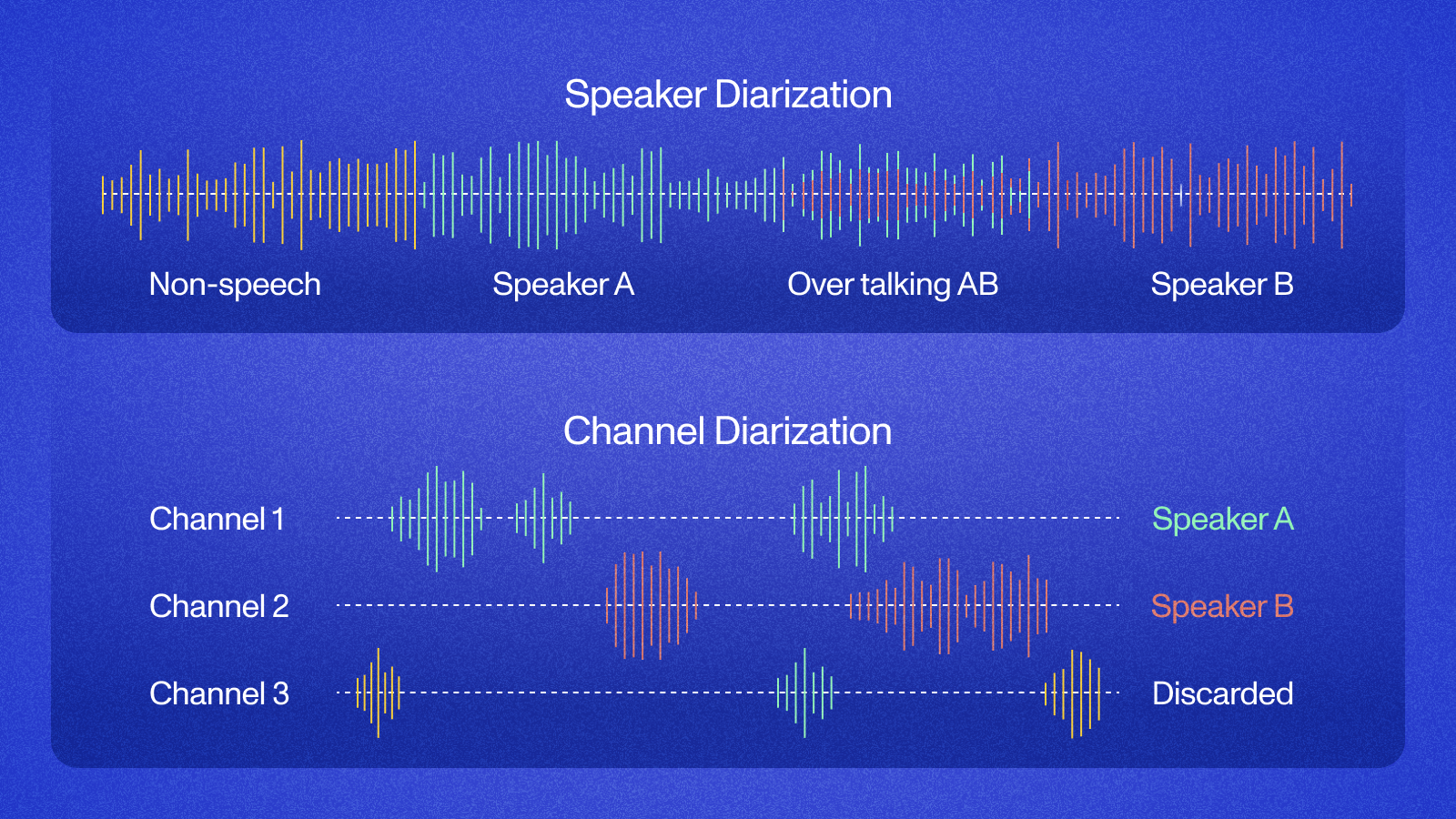
In this blog post, we’ll explain how Multichannel transcription and Speaker Diarization work, what their outputs look like, and how you can implement them using AssemblyAI.
Understanding Multichannel transcription
Multichannel transcription processes audio recordings with multiple separate channels, each capturing input from a distinct source, such as different speakers or devices. This approach isolates each participant’s speech, ensuring clarity and accuracy without overlap or confusion.
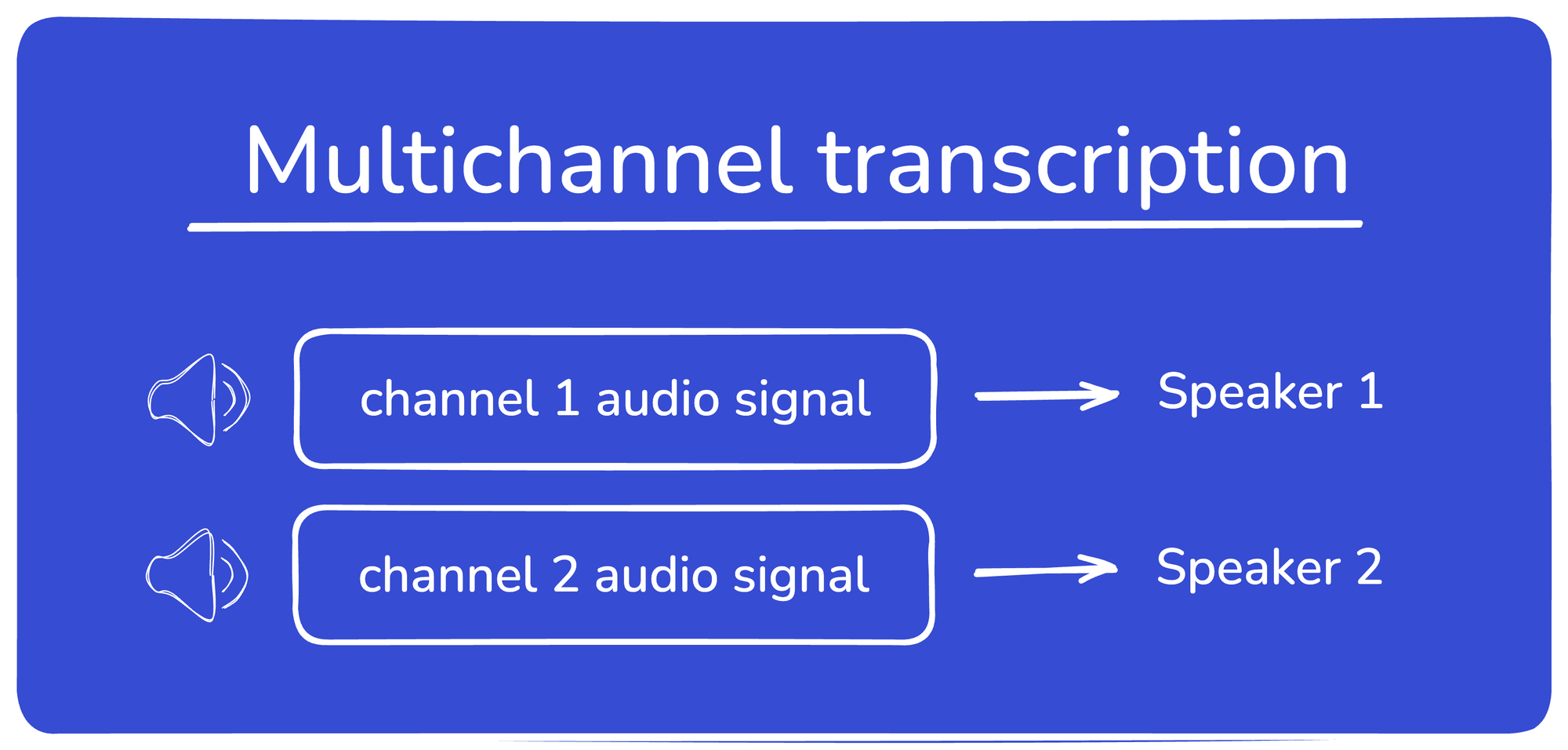
For instance, conference calls often record each participant’s microphone on a separate channel, making it easy to attribute speech to the correct person. In stereo recordings, which have two channels (left and right), Multichannel transcription can distinguish between the audio captured on each side, such as an interviewer on the left channel and an interviewee on the right. Similarly, podcast recordings may separate hosts and guests onto individual channels, and customer service calls often use one channel for the customer and another for the agent.
By keeping audio streams distinct, Multichannel transcription minimizes background noise, enhances accuracy, and provides clear speaker attribution. It simplifies the transcription process and delivers organized, reliable transcripts that are easy to analyze and use across various applications.
Understanding Speaker Diarization
Speaker Diarization is a more sophisticated process of identifying and distinguishing speakers within an audio recording, even when all voices are captured on a single channel. It answers the question: “Who spoke when?” by segmenting the audio into speaker-specific portions.

Unlike Multichannel transcription, where speakers are separated by distinct channels, diarization works within a single audio track to attribute speech segments to individual speakers. Advanced algorithms analyze voice characteristics such as pitch, tone, and cadence to differentiate between participants, even when their speech overlaps or occurs in rapid succession.
This technique is especially valuable in scenarios like recorded meetings, interviews, and panel discussions where speakers share the same recording track. For instance, a single-channel recording of a business meeting can be processed with diarization to label each participant’s speech, providing a structured transcript that makes conversations easy to follow.
By using Speaker Diarization, you can create clear and organized transcripts without the need for separate audio channels. This ensures accurate speaker attribution, improves usability, and allows for deeper insights into speaker-specific contributions in any audio recording.
Multichannel response
With AssemblyAI, you can transcribe each audio channel independently by configuring the multichannel
parameter. See how to implement it in the next section.
Here is an example JSON response for an audio file with two separate channels when multichannel
transcription is enabled:
{
"multichannel": true,
"audio_channels": 2,
"utterances": {
"text": "Here is Laura talking on channel one.",
"speaker": "1",
"channel": "1",
"start": ...,
"end": ...,
"confidence": ...,
"words": [
{
"text": "Here",
"speaker": "1",
"channel": "1"
"start": ...,
"end": ...,
"confidence": ...
},
...
]
},
{
"text": "And here is Alex talking on channel two.",
"speaker": "2",
"channel": "2",
"start": ...,
"end": ...,
"confidence": ...,
"words": [
{
"text": "And",
"speaker": "2",
"channel": "2"
"start": ...,
"end": ...,
"confidence": ...
},
...
]
}
}
The response contains the multichannel
field set to true,
and the audio_channels
field with the number of different channels.
The important part is in the utterances
field. This field contains an array of individual speech segments, each containing the details of one continuous utterance from a speaker. For each utterance, a unique identifier for the speaker
(e.g., 1, 2) and the channel
number are provided.
Additionally, the words
field is provided, containing an array of information about each word, again with speaker
and channel
information.
How to implement Multichannel transcription with AssemblyAI
You can use the API or one of the AssemblyAI SDKs to implement Multichannel transcription (see developer documentation).
Let's see how to use Multichannel transcription with the AssemblyAI Python SDK:
import assemblyai as aai
audio_file = "./multichannel-example.mp3"
config = aai.TranscriptionConfig(multichannel=True)
transcript = aai.Transcriber().transcribe(audio_file, config)
print(f"Number of audio channels: {transcript.json_response['audio_channels']}")
for utt in transcript.utterances:
print(f"Speaker {utt.speaker}, Channel {utt.channel}: {utt.text}")
To enable Multichannel transcription in the Python SDK, set multichannel
to True
in your TranscriptionConfig
. Then, create a Transcriber
object and call the transcribe
function with the audio file and the configuration.
When the transcription process is finished, we can print the number of audio channels and iterate over the separate utterances while accessing the speaker identifier, the channel, and the text of each utterance.
Speaker Diarization response
The AssemblyAI API also supports Speaker Diarization by configuring the speaker_labels
parameter. You’ll see how to implement it in the next section.
Here is an example JSON response for a monochannel audio file when speaker_labels
is enabled:
{
"multichannel": null,
"audio_channels": null,
"utterances": {
"text": "Today, I'm joined by Alex. Welcome, Alex!",
"speaker": "A",
"channel": null,
"start": ...,
"end": ...,
"confidence": ...,
"words": [
{
"text": "Today",
"speaker": "A",
"channel": null
"start": ...,
"end": ...,
"confidence": ...
},
...
]
},
{
"text": "I'm excited to be here!",
"speaker": "B",
"channel": null,
"start": ...,
"end": ...,
"confidence": ...,
"words": [
{
"text": "I'm",
"speaker": "B",
"channel": null
"start": ...,
"end": ...,
"confidence": ...
},
...
]
}
}
The response is similar to a Multichannel response, with an utterances
and a words
field including a speaker
label (e.g. “A”, “B”).
The difference to a Multichannel transcription response is that the speaker labels are denoted by “A”, “B” etc. rather than numbers, and that the multichannel
, the audio_channels
, and the channel
fields don’t contain values.
How to implement Speaker Diarization with AssemblyAI
Speaker Diarization is also supported through the API or one of the AssemblyAI SDKs (see developer documentation).
Here's how to implement Speaker Diarization with the Python SDK:
import assemblyai as aai
audio_file = "./monochannel-example.mp3"
config = aai.TranscriptionConfig(speaker_labels=True)
# or with speakers_expected:
# config = aai.TranscriptionConfig(speaker_labels=True, speakers_expected=2)
transcript = aai.Transcriber().transcribe(audio_file, config)
for utt in transcript.utterances:
print(f"Speaker {utt.speaker}: {utt.text}")
To enable Speaker Diarization in the Python SDK, set speaker_labels
to True
in your TranscriptionConfig
. Optionally, if you know the number of speakers in advance, you can improve the diarization performance by setting the speakers_expected
parameter.
Then, create a Transcriber
object and call the transcribe
function with the audio file and the configuration. When the transcription process is finished, we can again iterate over the separate utterances
while accessing the speaker label and the text of each utterance.
The code is similar to the Multichannel code example except for enabling speaker_labels
instead of multichannel
, and the result does not contain audio_channels
and channel
information.
How Speaker Diarization works
Speaker Diarization separates and organizes speech within a single-channel audio recording by identifying distinct speakers. This process relies on advanced algorithms and deep learning models to differentiate between voices, producing a structured transcript with clear speaker boundaries.
Here’s a high-level overview of the key steps in Speaker Diarization:
- Segmentation: The first step involves dividing the audio into smaller, time-based segments. These segments are identified based on acoustic changes, such as pauses, shifts in tone, or variations in pitch. The goal is to pinpoint where one speaker stops speaking, and another begins, creating the foundation for further analysis.
- Speaker Embeddings with Deep Learning models: Once the audio is segmented, each segment is processed using a deep learning model to extract speaker embeddings. Speaker embeddings are numerical representations that encode unique voice characteristics, such as pitch, timbre, and vocal texture.
- Clustering: After embeddings are extracted, clustering algorithms group similar embeddings into distinct clusters, with each cluster corresponding to an individual speaker. Both traditional clustering methods like K-means, or more advanced algorithms employing neural networks are common.
By following this process - segmentation, embedding generation, and clustering - speaker diarization can segment speech segments and attribute them to individual speakers.
Choosing between Multichannel and Speaker Diarization
Deciding between Multichannel transcription and Speaker Diarization depends on the structure of your audio and your specific needs. Both approaches are effective for separating and identifying speakers, but they are suited to different scenarios.
When to use Multichannel transcription
Multichannel transcription is ideal when your recording setup allows for distinct audio channels for each speaker or source. For example, conference calls, podcast recordings, or customer service calls often produce multichannel audio files. With each speaker recorded on a separate channel, transcription becomes straightforward, as there’s no need to differentiate speakers within a single track. Multichannel transcription ensures clarity, reduces overlap issues, and is particularly useful when high accuracy is required.
When to use Speaker Diarization
Speaker Diarization is the better choice for single-channel recordings where all speakers share the same audio track. This technique is commonly applied in scenarios like in-person interviews, panel discussions, or courtroom recordings. Diarization uses advanced algorithms to differentiate speakers, making it effective when you don’t have the option to record each participant on their own channel.
Making the right choice
If your recording setup supports separate channels for each speaker, Multichannel transcription is generally the more precise and efficient option.
However, if your audio is limited to a single channel or includes overlapping voices, Speaker Diarization is essential for creating structured and accurate transcripts.
Ultimately, the choice depends on the recording setup and the level of detail needed for the transcript.
Conclusion
Creating accurate and organized transcripts when multiple speakers are involved requires the right transcription method. In this post, we explored Multichannel transcription and Speaker Diarization, and how to use them with the AssemblyAI API.
Multichannel transcription is ideal for recordings with separate channels for each speaker, such as conference calls or podcasts. It ensures clear speaker attribution and eliminates overlap. With AssemblyAI, you use this feature by enabling the multichannel
parameter, allowing the API to process channels independently and provide structured, detailed transcripts.
Speaker Diarization works for single-channel recordings where all speakers share one track, such as interviews or meetings. By enabling the speaker_labels
parameter, AssemblyAI users Speaker Diarization and returns speech segments with a corresponding speaker label for each segment.
Understanding these methods and their API implementation helps you choose the best approach for your transcription needs, ensuring clarity, organization, and actionable results.
If you want to learn more about Multichannel transcription and Speaker Diarization, check out the following resources on our blog:
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.